Simple Calculator
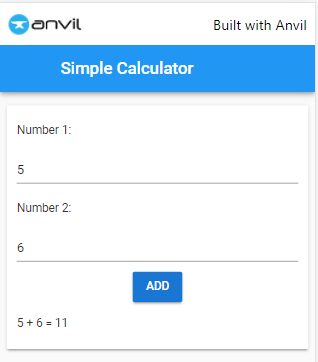
Simple Calculator Web App
Follow the steps to create a web app that can add two numbers. This will reenforce some concepts learned in the previous tutorial.
Go to Anvil and create a blank material-design app.
Name the project “Simple Calculator”.
Drag a label to where it says “Drop title here” and change the text to that label “Simple Calculator”.
Add a Card component to the layout. This will contain all of our UI elements.
Add Labels and TextBoxes to be able to input two numbers. Change the names of the TextBoxes to
self.number_1_box
andself.number_2_box
.Add a Button. Change the name to
self.calc_button
and change the text.Add a label below the button. Change the name to
self.result_label
. You can leave it without text.Add an event handler to the button. Make an alert() just to test it out.
In the event handler extract the text values from the two TextBoxes. Output the values in the alert to ensure you did it properly.
Set up a database table to be able to store a history of calculations. You can store the two numbers and the date this was done. This is silly, but it’s just good to practice.
Add a server module under the server code section and create a server-side function that will take the two numbers, then add a row to the database table. This function should return back the result of adding the two numbers together.
Back in the form, edit the button’s event handler function to call the server-side function. Don’t forget to sent it the two numbers. Also don’t forget to convert the values to integers! Save the return result in a variable and then use
alert()
to test if it sent back the proper result.Rather than show an alert for the result, use the
self.result_label
to display the result in a string somewhat approximating a message like"5 + 8 = 13"
Clear the input boxes when you press the button.
If you input a value that is not an integer, the program will crash. Fix this by using a
try/except
and make an alert pop up notifying the user of the invalid input.